Creating an app that adjusts to various screen sizes is essential for a good user experience. This post will discuss setting the minimum window size in a macOS SwiftUI app.
We’ll take inspiration from a dynamic window sizing example but provide a unique explanation focusing on the minimum dimensions.
Code Snippet for Minimum Window Size
Let’s consider the following code snippet for setting a minimum window size. This should be your entry point.
import SwiftUI
@main
struct HelloWorldApp: App {
var body: some Scene {
WindowGroup {
ContentView().frame(minWidth: 400, maxWidth: .infinity, minHeight: 300, maxHeight: .infinity)
}.windowResizability(.contentSize)
}
}
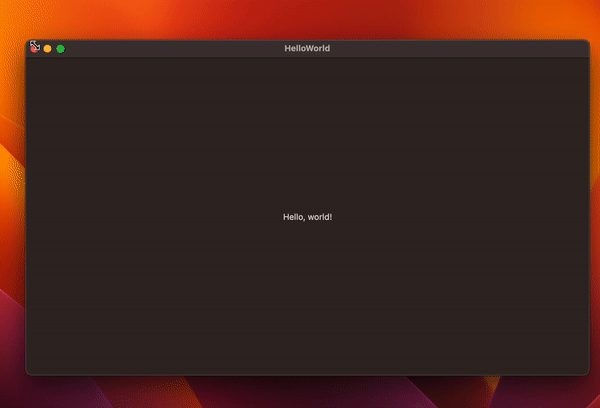
Code Explanation
Import SwiftUI
We start by importing SwiftUI, which provides us with all the tools we need for creating the app interface.
import SwiftUI
App Structure
We define an @main
structure that represents the main application. This structure conforms to the App
protocol.
@main
struct HelloWorldApp: App {
Define the Scene
Within the body
, we define a Scene
where our application’s main window resides. We use WindowGroup
to manage the app’s windows.
var body: some Scene {
WindowGroup {
Set the Frame
We define the frame dimensions inside ContentView
.
ContentView().frame(minWidth: 400, maxWidth: .infinity, minHeight: 300, maxHeight: .infinity)
Here, minWidth
and minHeight
are set to 400 and 300 respectively. This ensures that the user can’t resize the window below these dimensions. The maxWidth
and maxHeight
are set to .infinity
, letting users enlarge the window as much as they want.
Window Resizability
The .windowResizability(.contentSize)
sets how the window will respond to content changes. It restricts the window to resize according to the content size.
.windowResizability(.contentSize)
Setting the minimum window size in a SwiftUI macOS app can be achieved effortlessly with just a few lines of code. This feature ensures a consistent and user-friendly experience, avoiding any UI elements being too cramped or unreadable.