Setting up the right window size can make a world of difference in macOS apps. This guide will show you how to manage window sizes in a SwiftUI macOS application, focusing on two different examples.
Basics of WindowGroup in SwiftUI
WindowGroup
is the starting point for SwiftUI macOS apps. It controls the main window where your ContentView
is placed. While it provides some default settings, you can further tweak it to better control your window sizes.
@main
struct MyApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
Dynamic Window Size
In this example, the window has both minimum and maximum sizes. This allows for dynamic resizing by the user.
import SwiftUI
@main
struct HelloWorldApp: App {
var body: some Scene {
WindowGroup {
ContentView().frame(minWidth: 400, maxWidth: .infinity, minHeight: 300, maxHeight: .infinity)
}.windowResizability(.contentSize)
}
}
Here, the minWidth
is 400, and the minHeight
is 300. We set maxWidth
and maxHeight
to .infinity
, allowing the user to resize the window freely.
Fixed Window Size
In this example, the window size is fixed at 800×600 pixels and can’t be changed.
import SwiftUI
@main
struct HelloWorldApp: App {
var body: some Scene {
WindowGroup {
ContentView().frame(width: 800, height: 600)
}.windowResizability(.contentSize)
}
}
Here, we set the width
to 800 and the height
to 600, making the window size static.
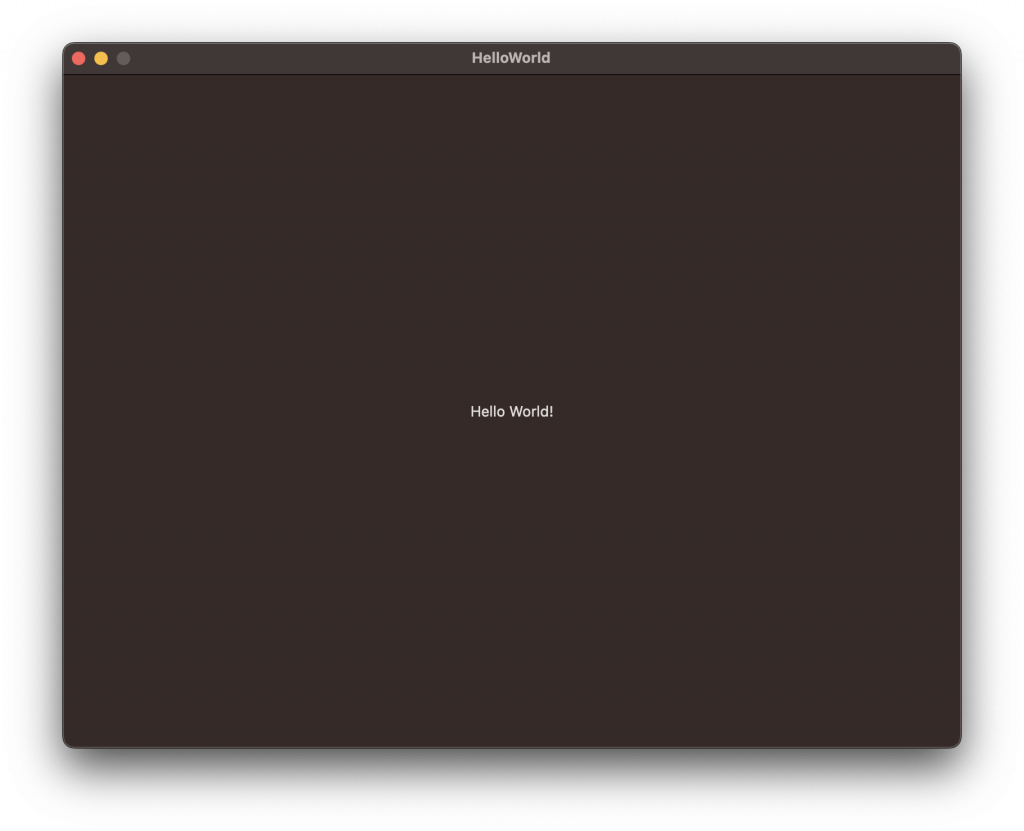
In both examples, you might have noticed .windowResizability(.contentSize)
. This method helps ensure that the window’s size matches the size of the content.
SwiftUI gives you multiple options for controlling window sizes in macOS applications. With just a few lines of code, you can either fix the window size or let users resize it according to their needs.
[…] take inspiration from a dynamic window sizing example but provide a unique explanation focusing on the minimum […]